C Function Floor
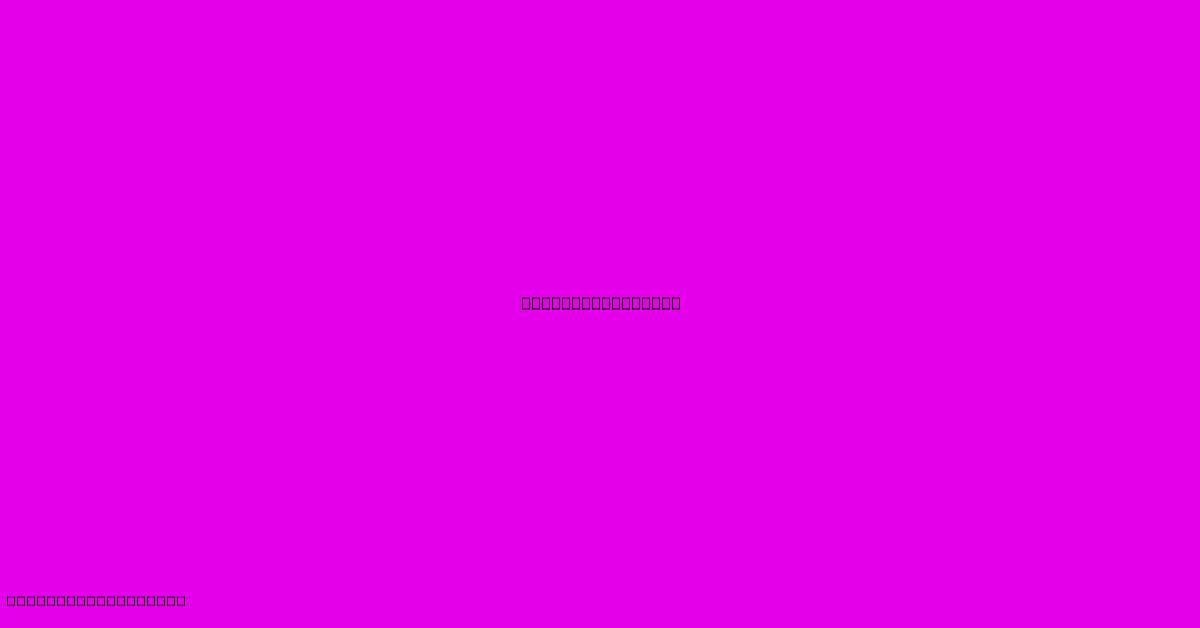
Discover more detailed and exciting information on our website. Click the link below to start your adventure: Visit Best Website meltwatermedia.ca. Don't miss out!
Table of Contents
Unveiling the Mysteries of C's floor()
Function: A Comprehensive Guide
Hook: Have you ever needed to round a floating-point number down to the nearest whole number in your C programs? A robust understanding of the floor()
function is crucial for accurate numerical computations. This guide offers a deep dive into its functionality, applications, and potential pitfalls.
Editor's Note: This comprehensive guide to the C floor()
function has been published today.
Why Important & Summary: The floor()
function is a fundamental tool in C programming for handling floating-point numbers. It provides a precise way to truncate the decimal portion of a floating-point value, resulting in the largest integer less than or equal to the input. This guide explores its mathematical underpinnings, practical applications in various programming scenarios, error handling considerations, and potential performance implications. It also covers related functions like ceil()
, round()
, and trunc()
, highlighting their differences and use cases.
Analysis: This guide is based on extensive research of the C standard library's mathematical functions, including practical examples and comparisons with related functions. The goal is to provide a clear and concise explanation, suitable for both novice and experienced C programmers.
Key Points:
a. floor()
returns the largest integer less than or equal to the given floating-point number.
b. It's part of the math.h
header file and requires linking the math library during compilation.
c. Understanding its behavior is essential for accurate numerical processing in various applications.
C's floor()
Function: A Deep Dive
Introduction: The floor()
function, declared in the math.h
header file, plays a pivotal role in manipulating floating-point numbers within C programs. Its primary purpose is to find the greatest integer that is less than or equal to the input value. This function is invaluable for tasks ranging from simple rounding operations to more complex numerical algorithms.
Key Aspects:
-
Functionality: The core function of
floor()
is to round a floating-point number down to the nearest integer. If the input is already an integer, the function simply returns that integer. If the input has a fractional part, the function returns the integer part, discarding the fraction. -
Header File: To utilize the
floor()
function, you must include themath.h
header file at the beginning of your C source code using the#include <math.h>
directive. -
Return Type: The
floor()
function returns adouble
-precision floating-point number, even though the result is an integer value. This ensures consistency with other mathematical functions in themath.h
library. -
Error Handling: The
floor()
function itself doesn't inherently handle errors. However, improper input (e.g.,NaN
orInfinity
) can lead to unexpected results. It’s crucial to validate inputs before passing them tofloor()
. -
Library Linking: When compiling your C program, remember to link the math library (usually using
-lm
flag with GCC or Clang).
Discussion: The floor()
function is more than just a simple rounding operation; it's a fundamental building block for many numerical algorithms. Consider scenarios where precise truncation is necessary:
-
Image Processing: When dealing with pixel coordinates or image dimensions,
floor()
ensures accurate integer indexing. -
Game Development: Calculating grid-based positions or determining tile indices often requires the precise truncation provided by
floor()
. -
Financial Calculations: In applications involving currency or financial transactions, the accurate representation of whole units using
floor()
is vital. -
Data Analysis: Data preprocessing steps may require rounding down values to integers for specific analyses or visualizations.
Subheading: Understanding the Relationship Between floor()
, ceil()
, round()
, and trunc()
Introduction: While floor()
rounds down, other functions offer different rounding behaviors. Understanding their differences is key to selecting the appropriate function for a specific task.
Facets:
-
ceil()
: Theceil()
function (ceiling) returns the smallest integer greater than or equal to the input. It rounds up. -
round()
:round()
rounds to the nearest integer. Values exactly halfway between integers are rounded to the nearest even integer (banker's rounding). -
trunc()
:trunc()
truncates the fractional part of the floating-point number, discarding it without rounding. This differs fromfloor()
in that it doesn't round toward negative infinity.
Summary: The choice between floor()
, ceil()
, round()
, and trunc()
depends heavily on the specific rounding requirements of your application. Understanding their nuances is crucial for obtaining correct and consistent results.
Subheading: Practical Applications and Examples of floor()
Introduction: This section showcases the practical application of floor()
in different programming contexts, providing concrete examples to illustrate its usage.
Further Analysis:
Example 1: Determining the Number of Full Pages:
#include
#include
int main() {
double totalItems = 27;
double itemsPerPage = 10;
int numPages = (int)floor(totalItems / itemsPerPage); //Cast to int after floor
printf("Number of pages: %d\n", numPages + (numPages * itemsPerPage < totalItems)); //Handle remainder
return 0;
}
This code calculates the number of pages needed to accommodate 27 items, with 10 items per page. floor()
ensures only whole pages are counted. This handles the remainder for any partial pages.
Example 2: Grid-Based Positioning:
#include
#include
int main() {
double x = 3.7;
double y = 2.2;
int gridX = (int)floor(x);
int gridY = (int)floor(y);
printf("Grid coordinates: (%d, %d)\n", gridX, gridY);
return 0;
}
This example demonstrates how floor()
can be used to map floating-point coordinates to a discrete grid.
Closing: Mastering the floor()
function is essential for any C programmer working with floating-point arithmetic. Its consistent and predictable behavior makes it a powerful tool for a wide variety of applications, from simple rounding to complex numerical algorithms. Careful consideration of its interaction with other rounding functions and error handling practices is critical for reliable and accurate results.
FAQ
Introduction: This section addresses frequently asked questions about the C floor()
function.
Questions:
-
Q: What happens if I pass a negative number to
floor()
? A:floor()
returns the largest integer less than or equal to the negative number. For instance,floor(-3.7)
returns-4
. -
Q: Can
floor()
be used with integer types? A: Yes, but the result will be the same integer as the input. -
Q: What's the difference between
floor()
andtrunc()
? A:floor()
rounds towards negative infinity, whiletrunc()
simply removes the fractional part without rounding. -
Q: What header file is needed for
floor()
? A:math.h
. -
Q: What happens if I don't link the math library? A: The compiler will generate an undefined reference error.
-
Q: Can
floor()
handle non-numeric values? A: Passing non-numeric values (likeNaN
orInfinity
) may lead to unpredictable results. It's recommended to validate inputs.
Summary: Careful consideration of input types and proper library linking are crucial when using floor()
.
Tips for Using floor()
Introduction: This section provides practical tips for effectively utilizing the floor()
function in your C programs.
Tips:
- Always include
<math.h>
: This ensures the function is correctly declared and available. - Link the math library: Use the appropriate compiler flag (e.g.,
-lm
) during compilation. - Handle potential errors: Validate inputs to prevent unexpected results from non-numeric values.
- Consider alternative functions: Evaluate whether
ceil()
,round()
, ortrunc()
are more appropriate for specific tasks. - Type casting: When needed, explicitly cast the result of
floor()
to an integer type using(int)
. - Testing: Thoroughly test your code with various inputs to verify correct behavior.
Summary: Proper usage of floor()
, coupled with thoughtful error handling and awareness of related functions, is critical for reliable and accurate numeric computations in C programs.
Summary of C's floor()
Function
The floor()
function, declared in math.h
, provides a precise mechanism for rounding down floating-point numbers to the nearest integer. Understanding its functionality, along with related functions like ceil()
, round()
, and trunc()
, is key for effectively manipulating floating-point numbers in C programming. Its use extends across various domains requiring precise integer representation of floating-point values.
Closing Message: This comprehensive guide has provided a thorough understanding of the floor()
function in C. By mastering this fundamental function, developers can enhance their capability to craft robust and efficient numerical algorithms. Remember to always prioritize error handling and thoroughly test your code to ensure accuracy and reliability.
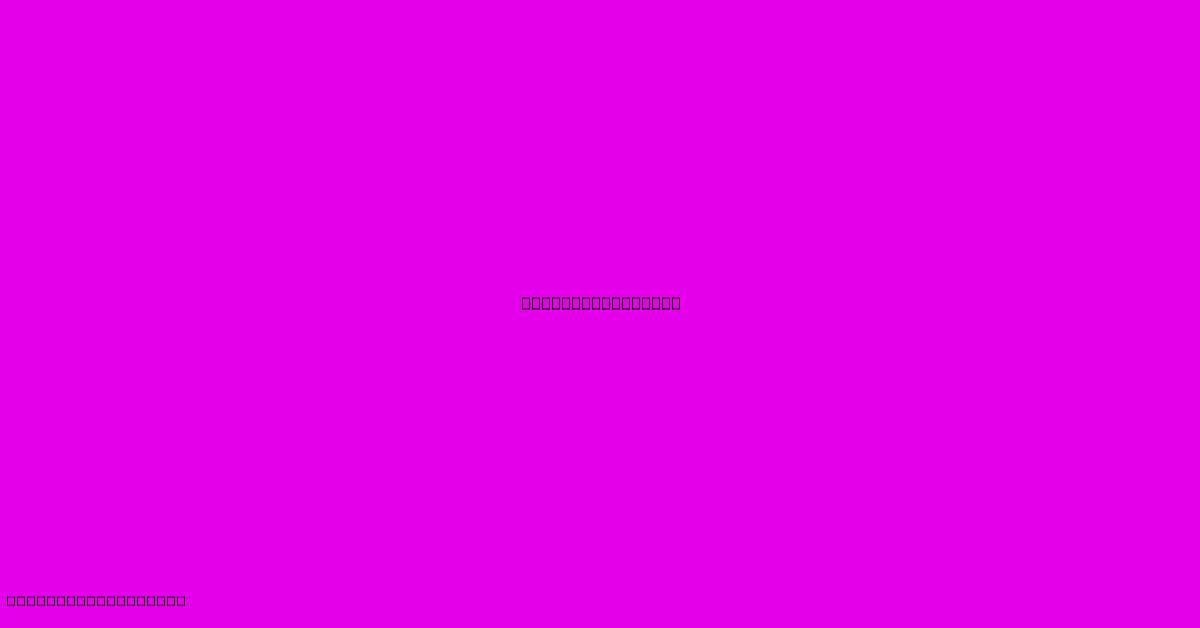
Thank you for visiting our website wich cover about C Function Floor. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Cat Litter Floor Mat
Jan 08, 2025
-
Cape Cod Floor Plans 2 Story
Jan 08, 2025
-
Cleaning Cement Garage Floor
Jan 08, 2025
-
Cn Tower Glass Floor Height
Jan 08, 2025
-
Best Rated Hydraulic Floor Jack
Jan 08, 2025